Hello again!
I got the new chip now and tried it but didnt get it to work, so I need some help in trouble-shooting.
The parts I use are:
Arduino UNO+5v
Sparkfun Levelconverter (LC):
https://www.sparkfun.com/products/8745?
Ap2-chip
Im doing the RX/TX switching between the UNO and the LC.
Im using the SW with the code above with a change, RX=#2 and TX=#3, and you can see the viring im using in the attached images.
Can you see what Im doing wrong?
The way I expect the code to work is to send a message over TX/RX the to chip and expect a answer in return, this is the right way how it should work, right?
In the vire-picture, red = 3.3, black = GND.
On the LC on the RX-line the level-converting are done by resistors, does this mean I have to use both TX lines (ch1 AND ch2) in the LC instead?
Is it correct to make the TX/RX switch between the UNO and LC or shall I do this between the LC and the AP2 Instead?
Going:
UNO TX-> LC TXO => LC TXI => AP2 RX
AP2 TX -> LC RXO => LC RXI => UNO TX
If a meassure on the TX / RX lines on the AP2 they have 1,8V resp 2.4V while "idle" can this be correct?
Thanks in advance and have a great weekend!
Image Attachments
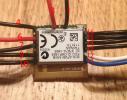
Click thumbnail to see full-size image